Custom Approval Triggers
Powered by RevOps Functions
Custom Approval Triggers combine the power of Approval Workflows and the power of RevOps functions to handle any part of your agreement approval process. The use of TypeScript allows for complete customization to validate your business requirements and RevOps will effortlessly handle your deployment and execution. This guide provides detailed instructions on how to implement this functionality effectively.
Custom Approval Triggers are a limited access beta feature. Please contact your Account Manager if you are interested in joining the beta. The information in this document is a work in progress and is subject to change.
Please provide any questions or feedback to support@revops.io or via your Slack Connect channel if applicable. Additional features and functionality will be added in the future.
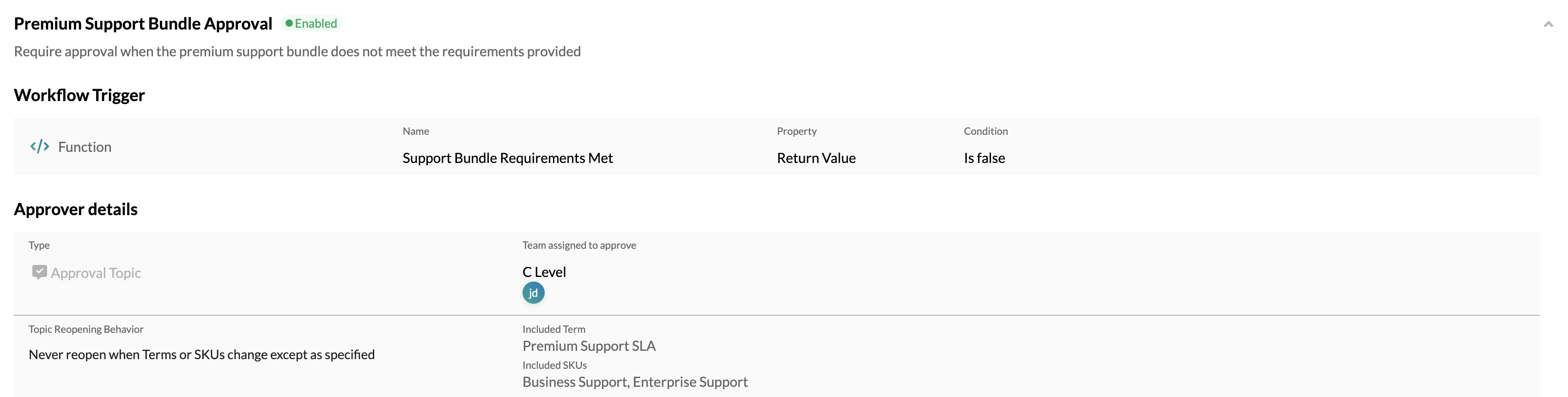
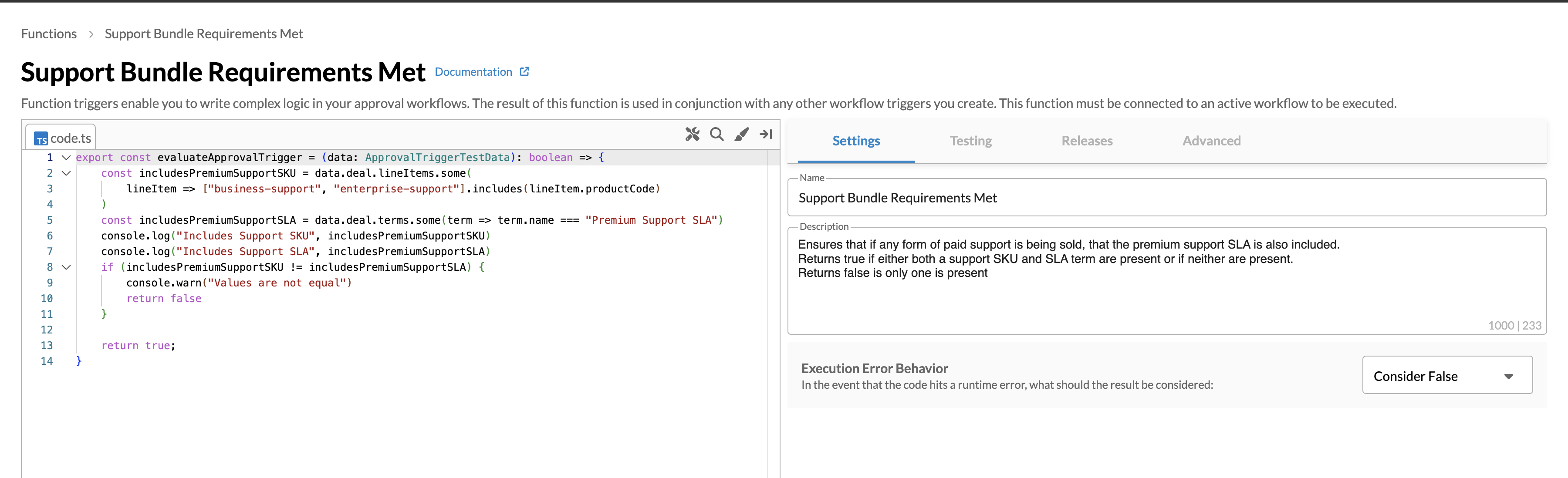
Getting Started
This guide covers specifics on the approval trigger function type. For more information about functions, visit RevOps Functions and review our Getting Started guide.
Basic Custom Trigger
A RevOps function is a named JavaScript/TypeScript function that is provided deal data and returns a boolean of true
or false
.
export const evaluateApprovalTrigger = (data: ApprovalTriggerTestData): boolean => {
const includesPremiumSupportSKU = data.deal.lineItems.some(
lineItem => ["business-support", "enterprise-support"].includes(lineItem.productCode)
)
const includesPremiumSupportSLA = data.deal.terms.some(term => term.name === "Premium Support SLA")
console.log("Includes Support SKU", includesPremiumSupportSKU)
console.log("Includes Support SLA", includesPremiumSupportSLA)
if (includesPremiumSupportSKU != includesPremiumSupportSLA) {
return false
}
return true;
}
In the example above, the function ensures the completeness of a SKU and Term dependency to ensure that if Business Support or Enterprise Support is sold, than the Premium Support SLA term is also included.
Function triggers can be combined with any other standard workflow trigger and can be referenced by multiple workflows.
Requirements and Details
- Function Name and Parameters
- Your code must export a function named
evaluateApprovalTrigger
. You can only export this function once. - This function will be invoked with a
data
parameter of typeApprovalTriggerTestData
containing all relevant attributes of terms and line items. - The function should return a boolean of
true
orfalse
. - Your function may also be
async
and return the appropriatePromise
. When utilizing promises, you should only ever successfully resolve the promise. Promises that are rejected will be treated as an execution error.
- Your code must export a function named
- Language Options
- RevOps Functions are powered by TypeScript (TS), which utilizes the same syntax as JavaScript (JS) with additional syntax for type checking. The Functions editor includes built in type definitions and type checking to highlight potential issues with your code.
- Users may choose to write in JavaScript and not include types, although we do not recommend it.
- Testing Functions
-
The Functions editor includes a section to test the result of your function based on a saved deal.
-
The tester will show you the results as would be sent to the workflow trigger evaluator and allow you to inspect the data passed to the function.
-
Output from
console.log
,console.warn
, andconsole.error
is automatically captured and made available in the tester and to admins during deal submission.
-
- Deal Submission Process
- When a user submits a deal for approval, your custom validation function will run alongside all other standard workflow operations.
- You can review the result of your function through Workflow Results along with any other standard result information. This also includes the option to view any console log output from the function.
Trigger Combinations
Function triggers can be combined with any other trigger and referenced in multiple workflows.
In the example below, the auto-approval workflow checks the deal terms, subtotal, and discount % using non-function triggers and checks the return value of two functions.
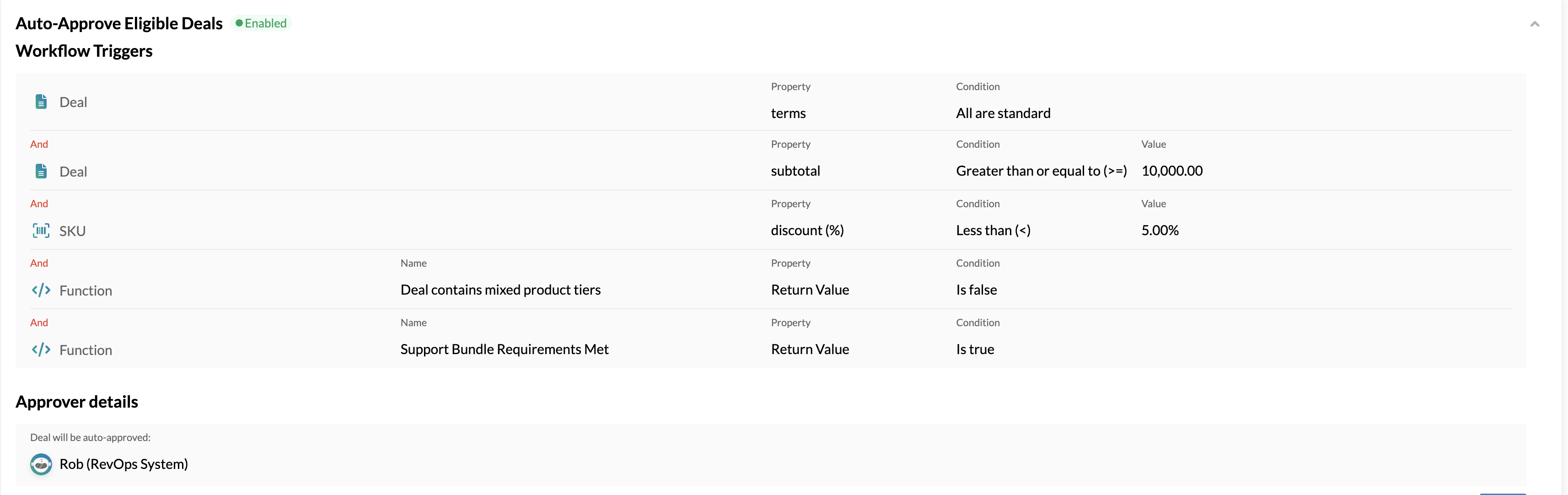
Each of those functions is then also referenced in a separate approval topic workflow checking for the inverse condition of the auto-approval result.
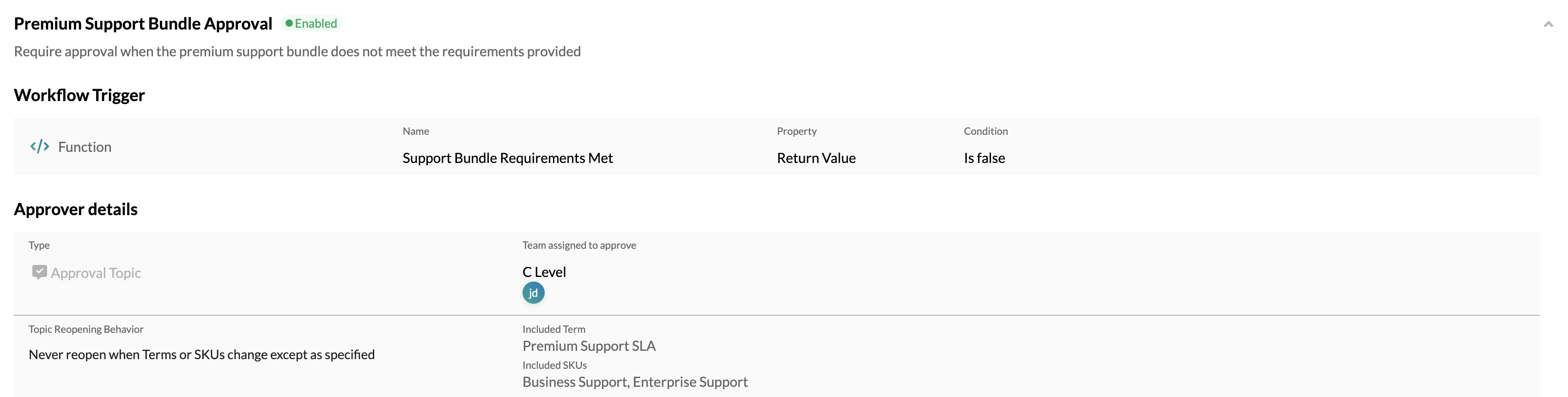
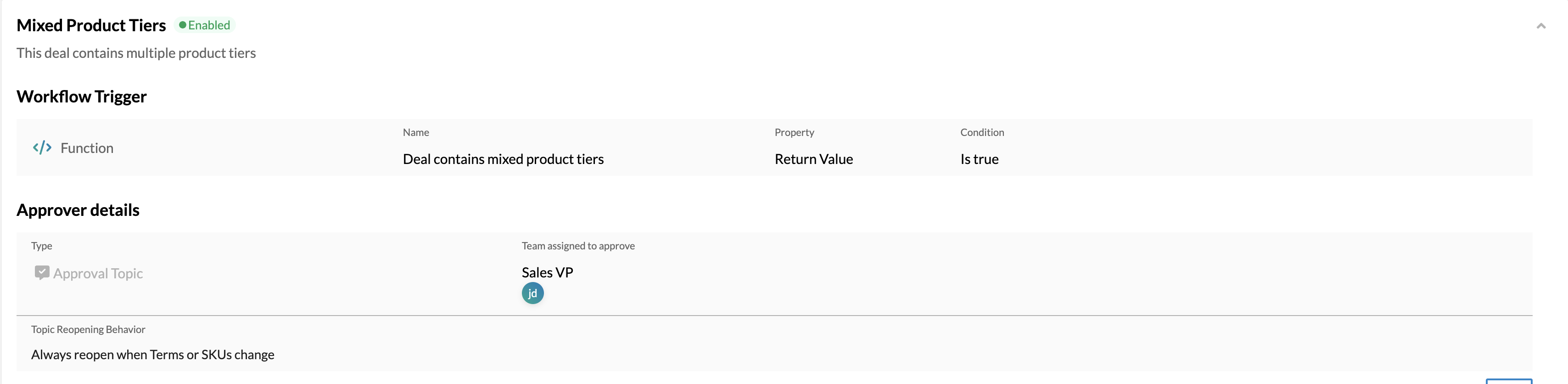
Function triggers are supported on any workflow type and can be used within approval levels.
Samples
A variety of samples can be found in Function Examples.
Error Behavior
In the event that your code results in an execution error or returns an unparsable value, RevOps will fallback to the value that you have configured.
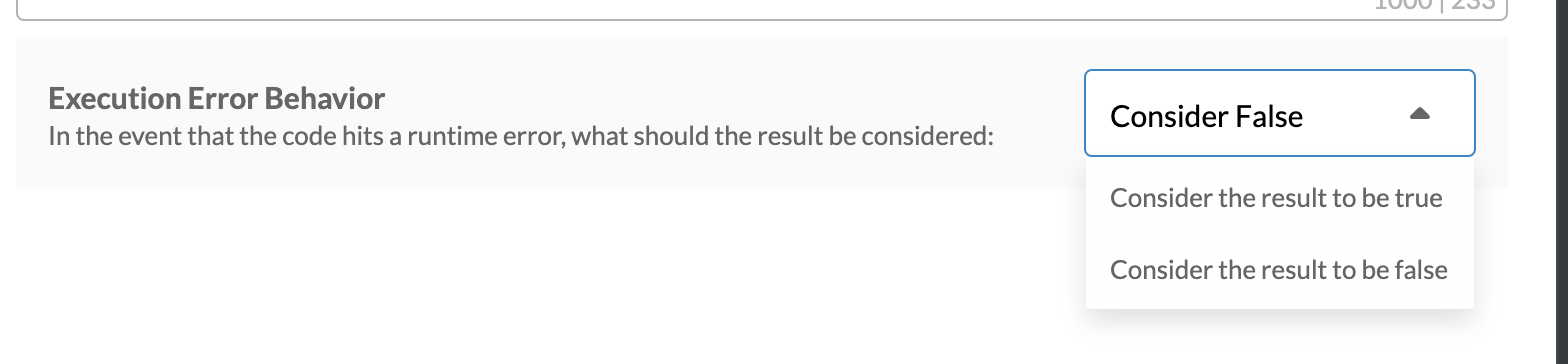
Boolean true
or false
are the only officially supported return types. However, any value that when stringified results in a case-insensitive form of true/false
, 1/0
, or y/n
will be converted to the appropriate boolean.
Type Definitions
Type information can be found in Type Definitions.
Additional Resources
Additional information about Functions, including its limitations and best practices, can be found in RevOps Functions.